Imperative paradigm
Focuses on creating statements that change program states by creating algorithms.Typically code will make use of conditional statements, loops and inheritance.
Imperative paradigm
↙ ↘
procedural language object-oriented programming
Ex: C, C++, PHP, Java, Assembly
code example in JavaScript :
class Number {
constructor
(number = 0) {
this.number = number;
}
add (x) {
this.number = this.number + x;
}
this.number = number;
}
add (x) {
this.number = this.number + x;
}
}
const myNumber = new Number (5);
myNumber.add (3);
console.log (myNumber.number); // 8
myNumber.add (3);
console.log (myNumber.number); // 8
Declarative paradigm
Focuses on building logic of software without actually describing its flow.
Focuses on building logic of software without actually describing its flow.
Declarative paradigm
↙ ↘
Logical function domain-specific languages
Ex: HTML, XML,CSS, Prolog, Haskell, F#, Lisp
Code example in JavaScript :
const sum = a => b => a
+ b;
console.log (sum (5) (3)); // 8
Programming paradigm is style of programming, a way of thinking about software construction.
Functional programming is a programming paradigm ,a style of building the structure and elements of computer programs, that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data.
Functional programming based on lambda calculus is Turing complete, avoids states, side effects and mutation of data.
Functional programming is about passing data from function to function to get a result.We can use functions as parameters, return them, build functions from other functions, and build customer functions.
Procedural programming is a programming paradigm, derived from structured programming, based upon the concept of the procedure call. Procedures, also known as routines, subroutines, or functions (not to be confused with mathematical functions, but similar to those used in functional programming), simply contain a series of computational steps to be carried out.
Procedural programming, also known as inline programming takes a top-down approach.It is about writing a list of instructions to tell the computer what to do step by step.It relies on procedures or routines.
Functional programming
Functional programming is a programming paradigm ,a style of building the structure and elements of computer programs, that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data.
Functional programming based on lambda calculus is Turing complete, avoids states, side effects and mutation of data.
Functional programming is about passing data from function to function to get a result.We can use functions as parameters, return them, build functions from other functions, and build customer functions.
Procedural programming
Procedural programming is a programming paradigm, derived from structured programming, based upon the concept of the procedure call. Procedures, also known as routines, subroutines, or functions (not to be confused with mathematical functions, but similar to those used in functional programming), simply contain a series of computational steps to be carried out.
Procedural programming, also known as inline programming takes a top-down approach.It is about writing a list of instructions to tell the computer what to do step by step.It relies on procedures or routines.
Lambda Calculus
- Notation for functions, free and bound variables.
- Calculate using substitution, rename and avoid capture.
E :: = x(variables)
| E1 E2(function application)
| λx.E(function creation)
Where λx.E is called Lambda abstraction and E is known as λ-expressions.
Here, we can’t start with '+' because it only operates on numbers. There are two reducible expressions: (* 5 6) and (* 8 3).
This is called β-reduction.
When there are multiple terms, we can handle them as follows
In an expression, each appearance of a variable is either "free" (to λ) or "bound" (to a λ).
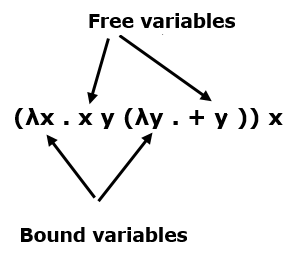
Alpha reduction is very simple and it can be done without changing the meaning of a lambda expression.
For example
The Church-Rosser Theorem states the following
Evaluating Lambda Calculus
Pure lambda calculus has no built-in functions. Let us evaluate the following expression
(+ (* 5 6) (* 8 3))
Here, we can’t start with '+' because it only operates on numbers. There are two reducible expressions: (* 5 6) and (* 8 3).
We can reduce either one first. For example
(+ (* 5 6) (* 8 3)) (+ 30 (* 8 3)) (+ 30 24) = 54
β-reduction Rule
We need a reduction rule to handle λs
(λx . * 2 x) 4
(* 2 4)
= 8
This is called β-reduction.
The formal parameter may be used several times
(λx . + x x) 4
(+ 4 4)
= 8
When there are multiple terms, we can handle them as follows
(λx . (λx . + (− x 1)) x 3) 9
The inner x belongs to the inner λ and the outer x belongs to the outer one.
(λx . + (− x 1)) 9 3 + (− 9 1) 3 + 8 3 = 11
Free and Bound Variables
In an expression, each appearance of a variable is either "free" (to λ) or "bound" (to a λ).
β-reduction of (λx . E) y replaces every x that occurs free in E with y. For Example
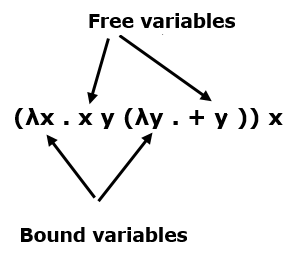
Alpha Reduction
Alpha reduction is very simple and it can be done without changing the meaning of a lambda expression.
λx . (λx . x) (+ 1 x) ↔ α λx . (λy . y) (+ 1 x)
For example
(λx . (λx . + (− x 1)) x 3) 9 (λx . (λy . + (− y 1)) x 3) 9 (λy . + (− y 1)) 9 3 + (− 9 1) 3 + 8 3 11
Church-Rosser Theorem
The Church-Rosser Theorem states the following
- If E1 ↔ E2, then there exists an E such that E1 → E and E2 → E. “Reduction in any way can eventually produce the same result.”
- If E1 → E2, and E2 is normal form, then there is a normal-order reduction of E1 to E2. “Normal-order reduction will always produce a normal form, if one exists.”
Lambda Expression
Ex : Smalltalk, Lisp, Ruby, Scala, Python, Java, JavaScript
Code example in Python :
square = lambda x: x**2
square(6) //-> 36
"Referential Transparency" and "no side- effects" in functional programming.
A function that returns always the same result for the same input is called a pure function. A pure function, therefore, is a function with no observable side effects, if there are any side effects on a function the evaluation could return different results even if we invoke it with the same arguments. We can substitute a pure function with its calculated value.
The ability to replace an expression with its calculated value is called referential transparency.
Ex: def f(x: Int, y: Int) = x + y
for the input
f(4, 4)
can be replaced by
8
The importance of referential transparency is that it allows the programmer and the compiler to reason about program behavior as a rewrite system. This can help in proving correctness, simplifying an algorithm, assisting in modifying code without breaking it, or optimizing code by means of memoization, common subexpression elimination, lazy evaluation, or parallelization.
A side effect refers simply to the modification of some kind of state-of instance :
- Changing the value of a variable.
- Writing or disabling a button in the user interface.
Functional programming is based on the simple premise that your functions should not have side effects; they are considered evil in this paradigm. If a function has side effects we call it a procedure, so functions do not have side effects. We consider that a function has a side effect if it modifies a mutable data structure or variable, uses IO, throws an exception or halts an error; all of these things are considered side effects.
The reason why side effects are bad is because, if you had them, a function can be unpredictable depending on the state of the system; when a function has no side effects we can execute it anytime, it will always return the same result, given the same input.
The reason why side effects are bad is because, if you had them, a function can be unpredictable depending on the state of the system; when a function has no side effects we can execute it anytime, it will always return the same result, given the same input.
This paradigm doesn't try to get rid of side effects, just limit them when they are required. Side effects are needed because without them our programs will do only calculations. We often have to write to databases, integrate with external systems or write files.
Key Features of Object Oriented Programming
Object Oriented programming is method of implementation in which programs are organized as a collection of objects which cooperative to solve a problem.
- A complex system is developed using smaller sub systems.
- Sub systems are independent units containing their own data and functions.
- Can reuse these independent units to solve many different problems.
A class is the abstract definition of the data type.
Ex: thing, person or something that is imaginary
An object is a specific instance of the data type(class).
OOP Concepts
- Abstraction
- Encapsulation
- Polymorphism
- Inheritance
- Association
- Composition
- Aggregation
Abstraction
Abstraction is the concept of hiding the internal details and describing things in simple terms.
There are many ways to achieve abstraction in object-oriented programming.Such as encapsulation and inheritance.
Encapsulation
It is the process of grouping related attributes and methods together, giving a name to the unit and providing an interface for outsiders to communicate with the unit.
Encapsulation is the technique used to implement abstraction in object-oriented programming.Encapsulation is used for access restriction to class members and methods.
Access modifier keywords are used for encapsulation in object oriented programming.
Ex: Private, Protected, public
Class Student {
private int id;
private String name;
public void setId(int id){
this.id= id;
}
public int getId(){
return id;
}
public void setName(String name){
this.name = name;
}
public String getName(){
return name;
}
}
Polymorphism
Polymorphism is the concept where an object behaves differently in different situations.There are two types of polymorphism.
- compile time polymorphism
- runtime polymorphism
Compile time polymorphism is achieved by method overloading.
public class Circle {
public void draw(){
System.out.println("Drawing circle with default color Black and diameter 1 cm.");
}
public void draw(int diameter){
System.out.println(Drawing circle with default color Black and diameter" + diameter+ "cm.");
}
public void draw(int diameter, String color){
System.out.println("Drawing circle with color"+color+"and"+diameter+"cm.");
}
}
Runtime polymorphism is achieved by the method overriding.
public interface Shape{
public void draw();
}
public class Circle implements Shape{
@Override
public void draw(){
System.out.println("Drawing Circle");
}
}
public class Square implements Shape{
@Override
public void draw(){
System.out.println("Drawing Square"):
}
}
Shape is the Superclass and there are two subclasses Circle and Square.
Shape sh = new Circle();
sh.draw();
Shape sh1 = getShape();
sh1.draw();
Inheritance
The object that is getting inherited is called super class and the object that inherits the superclass called subclass.
We use "extends" keyword in java to implement inheritance.
class SuperClassA{
public void foo(){
System.out.println("SuperClassA");
}
}
class SubClassB extends SuperClassA{
public void bar(){
System.out.println("SubClassB");
}
}
public class Test{
public static void main(String args[]){
SubClassB a = new SubClassB();
a.foo();
a.bar();
}
}
Association
Association is the OOP concept to define the multiplicity between objects.
Aggregation
Aggregation is a special type of association.In aggregation objects have their own life cycle but there is an ownership.
Composition
Composition is a special type of association.Composition is a more restrictive form in aggregation.
Comparison of programming paradigms
There are two main approaches to programming :
- Imperative programming – focuses on how to execute, defines control flow as statements that change a program state.
- Declarative programming – focuses on what to execute, defines program logic, but not detailed control flow.
The following are widely considered the main programming paradigms, as seen when measuring programming language popularity:
- Procedural programming, structured programming – specifies the steps a program must take to reach a desired state.
- Functional programming – treats programs as evaluating mathematical functions and avoids state and mutable data
- Object-oriented programming (OOP) – organizes programs as objects: data structures consisting of data fields and methods together with their interactions.
The following are common types of programming that can be implemented using different paradigms:
- Event-driven programming – program control flow is determined by events, such as sensor inputs or user actions (mouse clicks, key presses) or messages from other programs or threads.
- Automata-based programming – a program, or part, is treated as a model of a finite state machine or any other formal automaton.
Difference between Compiled languages, Scripting languages, and Markup languages
Programming languages are those that their end results are compiled. You most certainly use IDEs (Integrated Development Environments) to make use of these languages. If u think about it their examples are as follows : Java, visual basic, C, C++, C#, etc.
Markup languages are languages that are not in any way executed or used to perform actions but they are used to structure data, identify data or present data as the case may be.
EX :
- HTML(defines web content)
- XHTML(same with html but with some differences)
- XML(for structuring data, in some cases for defining UI structure as its used in Android app development), etc.
Scripting languages are languages that are not compiled, more like interpreted at run-time. It's like a file containing instructions for a computer to follow to carry out a task. Think of it like this. If you were to feature in a movie, u will definitely be given a script to read, memorize and follow. Hence the script becomes your guide to perform your roles in that movie.It's as simple as that.
Ex: javascript, VB script, Perl, python, php, she'll script, etc
Role of the Virtual Runtime Machines
The virtual machine function is a function for the realization of virtual machine environment. This function enables you to create multiple independent virtual machines on one physical machine by virtualizing resources such as the CPU, memory, network and disk that are installed on a physical machine.
Advances in JavaScript Performance
Let's understand this with the help of an example: As soon as the above code loads into the browser, JS engine pushes global execution context in the execution context stack.When all the code is executed JS engines pops out the global execution context and execution of JavaScript ends.
A program such as C++ or Java needs to be compiled before it is run. The source code is passed through a program called a compiler, which translates it into bytecode that the machine understands and can execute. ... Instead, an interpreter in the browser reads over the JavaScript code, interprets each line, and runs it.
The way of output of an HTML document is rendered
HTML (Hypertext Markup Language) is a web-based scripting language. It is mainly used to structure the look and function of websites. Any file containing HTML code is saved using the extension ".HTML". All modern browsers -- such as Google Chrome, Safari and Mozilla Firefox -- recognize this format and can open these files, so all you need to do to run an HTML file is open it in your Web browser of choice.
If we use this HTML code,
<div class="st_view recipe-tab ingredients st_view_first st_view_active" style="position: absolute; left: 0px;">
<h1 class="tab-hint">
Yields: <span itemprop="recipeYield" class="tab-hint-value">2 Servings</span>
</h1>
<ol>
<li itemprop="ingredients">1 <strong> Banana Nut Muffin Bar</strong>
</li>
<li itemprop="ingredients">3 tablespoons <strong>Vanilla Milkshake Protein Powder</strong>
</li>
<li itemprop="ingredients">1
<sup>1</sup>⁄
<sub>2</sub> tablespoons banana, mashed
</li>
<li itemprop="ingredients">
<sup>1</sup>⁄
<sub>2</sub> tablespoon unsweetened almond milk
</li>
<li itemprop="ingredients">1 teaspoon walnuts, crushed
</li>
<li itemprop="ingredients">
<sup>1</sup>⁄
<sub>4</sub> teaspoon banana extract
</li>
<li itemprop="ingredients">
<sup>1</sup>⁄
<sub>4</sub> teaspoon zero-calorie sweetener
</li>
<li itemprop="ingredients">Pinch of cinnamon
</li>
</ol>
This renders the following output.
CASE Tools
- Computer-Aided Software Engineering(CASE) tools are automated software packages that help to automate activities in the SDLC.
- CASE tools aim to enforce an engineering-type approach to the development of software system.
- CASE tools range from simple diagramming tools to very sophisticated program to document and automate most of the stages in the SDLC.
- CASE tools used since the early 1990s.
CASE Workbenches
- A coherent set of tools that is designed to support related software process activities such a analysis, design or testing.
- Analysis and design workbenches support system modelling during both requirements engineering and system design.
- These workbenches may support for a several different types of system model.
CASE Environments
A reference model for CASE (computer-aided software engineering) environments identifies five sets of services that such an environment should provide. It should also provide ‘plug-in’ facilities for individual CASE tools that use these services. A diagram of this model is shown in Figure 1.
Figure 1. The CASE environment reference model
The five levels of service in the CASE reference model are:
- Data repository services These provide facilities for the storage and management of data items and their relationships.
- Data integration services These provide facilities for managing groups or the establishment of relationships between them. These services and data repository services are the basis of data integration in the environment.
- Task management services These provide facilities for the definition and enactment of process models. They support process integration.
- Message services These provide facilities for tool-tool, environment-tool and environment-environment communications. They support control integration.
- User interface services These provide facilities for user interface development. They support presentation integration.
This reference model tells us what might be included in any particular CASE environment, although it is important to emphasise that not every feature of a reference architecture will be included in actual architectural designs. It means that we can ask questions of a system such as ‘how are the data repository services provided?’ and ‘does the system provide task management?’
Again, the principal value of this reference architecture is as a means of classifying and comparing integrated CASE tools and environments. In addition, it can also be used in education to highlight the key features of these environments and to discuss them in a generic way.
Software Framework
A software framework is an abstraction in which software providing generic functionality can be selectively changed by additional user-written code, thus providing application-specific software.
A software framework provides a standard way to build and deploy applications.
Software Library
A software library is a suite of data and programming code that is used to develop software programs and applications. It is designed to assist both the programmer and the programming language compiler in building and executing software.
Software Plugins
A plugin is a software add-on that is installed on a program, enhancing its capabilities.For example, if you wanted to watch a video on a website, you may need a plugin to play it because your browser installed on a program, enhancing its capabilities.For example, if you wanted to watch a video on a website, you may need a plugin to play it because your browser doesn't have the tools it needs. You can think of it like getting a Blu-ray player for your Blu-ray disc.
References :
https://stackoverflow.com/questions/42335548/get-rendered-html-output
https://www.quora.com/What-is-the-difference-between-programming-languages-markup-languages-and-scripting-languages-in-terms-of-how-they-manipulate-create-use-data-What-are-the-differences
https://en.wikipedia.org/wiki/Comparison_of_programming_paradigms
https://www.journaldev.com/12496/oops-concepts-java-example
https://medium.com/front-end-weekly/imperative-versus-declarative-code-whats-the-difference-adc7dd6c8380
https://www.tutorialspoint.com/functional_programming/functional_programming_lambda_calculus.htm
https://www.wikihow.com/Run-a-HTML-File
https://www.computerhope.com/jargon/p/plugin.htm
https://www.techopedia.com/definition/3828/software-library
https://en.wikipedia.org/wiki/Software_framework